So recently, because of my video post on making DIY amiibo (https://youtu.be/d2MAynHZsiU), I’ve gained a few extra followers / subscribers. So I thought to myself, maybe I can take the components that I have laying around and build a DIY Sub counter. The experience was actually extremely easy!! The only things you need are few key components. So let’s get started.
Required Hardware
NodeMCU v1.0
LCD I2C 16×2
Jumper Wires
Breadboard
Setup Ardurino IDE with Node MCU 1.0
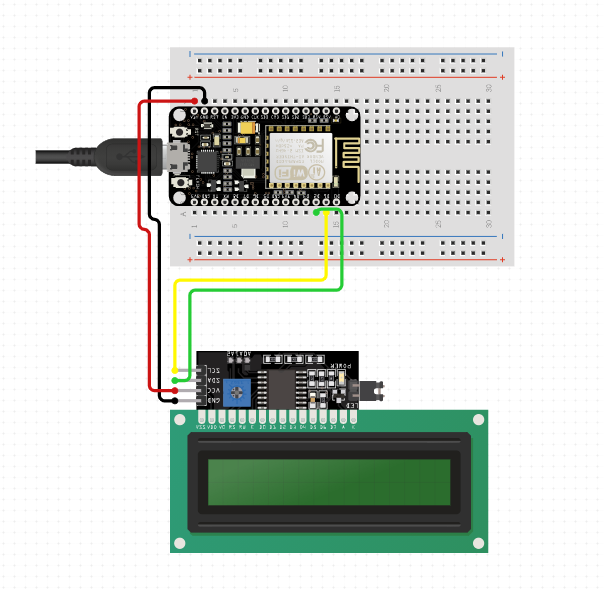
Put in the follow code and make sure you edit the following:
/*******************************************************************
YT channel Stats for NodeMCU with 16x2 LCD I2C
03/16/2021 v0.0001
by Min-Hsao Chen
Based on
ChannelStatistics by
Written by Brian Lough
https://github.com/sponsors/witnessmenow/
and
Arduino Gadgest: Youtube Subscribers Counter
https://www.hackster.io/thearduinoworld/arduino-gadgets-youtube-subscribers-counter-49f125#
*******************************************************************/
// ----------------------------
// Standard Libraries
// ----------------------------
#include <ESP8266WiFi.h>
#include <WiFiClientSecure.h>
#include <LiquidCrystal_I2C.h>
// ----------------------------
// Additional Libraries - each one of these will need to be installed.
// ----------------------------
#include <YoutubeApi.h>
// Library for connecting to the Youtube API
// Search for "youtube" in the Arduino Library Manager
// https://github.com/witnessmenow/arduino-youtube-api
#include <ArduinoJson.h>
// Library used for parsing Json from the API responses
// Search for "Arduino Json" in the Arduino Library manager
// https://github.com/bblanchon/ArduinoJson
//------- Replace the following! ------
char ssid[] = "xyz"; // !!! replace xyz with your network SSID Wifi (name)
char password[] = "xyz"; // !!! replace xyz with your network key Wifi
//--- Google Youtube API ----
#define API_KEY "xyz" // replace xyz with your google apps API Token
#define CHANNEL_ID "xyz" // replace xyz with your youtube channel ID
//------- ---------------------- ------
WiFiClientSecure client;
YoutubeApi api(API_KEY, client);
LiquidCrystal_I2C lcd(0x27, 16, 2);
// Define youtube icon
// The following creates the youtube play logo on 16x2 LCD
byte YT1[8] =
{
0b00011,
0b00111,
0b01111,
0b11111,
0b11111,
0b11111,
0b11111,
0b11111
};
byte YT2[8] =
{
0b11111,
0b11111,
0b11011,
0b11001,
0b11000,
0b11000,
0b11000,
0b11000
};
byte YT3[8] =
{
0b11111,
0b11111,
0b11111,
0b11111,
0b11111,
0b01111,
0b00111,
0b00011
};
byte YT4[8] =
{
0b11000,
0b11100,
0b11110,
0b11111,
0b11111,
0b11111,
0b11111,
0b11111
};
byte YT5[8] =
{
0b11000,
0b11000,
0b11000,
0b11000,
0b11001,
0b11011,
0b11111,
0b11111
};
byte YT6[8] =
{
0b11111,
0b11111,
0b11111,
0b11111,
0b11111,
0b11110,
0b11100,
0b11000
};
// timeBetweenRequests is the time between request 60000 ms = 60s
unsigned long timeBetweenRequests = 60000;
unsigned long nextRunTime;
long subs = 0;
int ytsubcount;
void setup() {
Serial.begin(115200);
// Set WiFi to station mode and disconnect from an AP if it was Previously
// connected
WiFi.mode(WIFI_STA);
WiFi.disconnect();
delay(100);
// Attempt to connect to Wifi network:
Serial.print("Connecting Wifi: ");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
Serial.print(".");
delay(500);
}
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
IPAddress ip = WiFi.localIP();
Serial.println(ip);
// Required if you are using ESP8266 V2.5 or above
client.setInsecure();
// If you want to enable some extra debugging
api._debug = true;
// initiate LCD
lcd.begin();
lcd.clear();
}
void loop() {
if (millis() > nextRunTime) {
if(api.getChannelStatistics(CHANNEL_ID))
{
Serial.println("---------Stats---------");
Serial.print("Subscriber Count: ");
ytsubcount=api.channelStats.subscriberCount;
Serial.println(ytsubcount);
Serial.print("View Count: ");
Serial.println(api.channelStats.viewCount);
Serial.print("Video Count: ");
Serial.println(api.channelStats.videoCount);
// Probably not needed :)
//Serial.print("hiddenSubscriberCount: ");
//Serial.println(api.channelStats.hiddenSubscriberCount);
Serial.println("------------------------");
// LCD output
lcd.createChar(1,YT1);
lcd.createChar(2,YT2);
lcd.createChar(3,YT3);
lcd.createChar(4,YT4);
lcd.createChar(5,YT5);
lcd.createChar(6,YT6);
lcd.setCursor(0,0);
lcd.write(1);
lcd.setCursor(1,0);
lcd.write(2);
lcd.setCursor(2,0);
lcd.write(3);
lcd.setCursor(3,0);
lcd.write(4);
lcd.setCursor(0,1);
lcd.write(3);
lcd.setCursor(1,1);
lcd.write(5);
lcd.setCursor(2,1);
lcd.write(1);
lcd.setCursor(3,1);
lcd.write(6);
lcd.setCursor(5,0);
//--- Replace the following print lines Keep it under 11 char combined
//--- currently Min's YT
lcd.print("Min's YT");
//--- Second Line Text... current is Sub:
lcd.setCursor(5,1);
lcd.print("Sub:");
lcd.setCursor(9,1);
lcd.print(ytsubcount);
}
nextRunTime = millis() + timeBetweenRequests;
}
}
You will need to have your Google API information. Take a look at this youtube videos for details.
If you don’t know it, you can find your own YouTube channel ID here. See here for additional detail on the ardurino youtube API library (GitHub – witnessmenow/arduino-youtube-api: A wrapper around the youtube api for arduino).